mirror of
https://git.bsd.gay/fef/nyastodon.git
synced 2024-11-02 01:21:11 +01:00
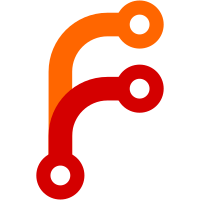
This PR adds a new notification cleaning mode, super perfectly tuned for accessibility, and removes the previous notification cleaning functionality as it's now redundant. * w.i.p. notif clearing mode * Better CSS for selected notification and shorter text if Stretch is off * wip for rebase ~ * all working in notif clearing mode, except the actual removal * bulk delete route for piggo * cleaning + refactor. endpoint gives 422 for some reason * formatting * use the right route * fix broken destroy_multiple * load more notifs after succ cleaning * satisfy eslint * Removed CSS for the old notif delete button * Tabindex=0 is mandatory In order to make it possible to tab to this element you must have tab index = 0. Removing this violates WCAG and makes it impossible to use the interface without good eyesight and a mouse. So nobody with certain mobility impairments, vision impairments, or brain injuries would be able to use this feature if you don't have tabindex=0 * Corrected aria-label Previous label implied a different behavior from what actually happens * aria role localization & made the overlay behave like a checkbox * checkboxes css and better contrast * color tuning for the notif overlay * fanceh checkboxes etc and nice backgrounds * SHUT UP TRAVIS
82 lines
1.8 KiB
JavaScript
82 lines
1.8 KiB
JavaScript
// Package imports //
|
|
import React from 'react';
|
|
import ImmutablePropTypes from 'react-immutable-proptypes';
|
|
import ImmutablePureComponent from 'react-immutable-pure-component';
|
|
|
|
// Mastodon imports //
|
|
|
|
// Our imports //
|
|
import StatusContainer from '../status/container';
|
|
import NotificationFollow from './follow';
|
|
|
|
export default class Notification extends ImmutablePureComponent {
|
|
|
|
static propTypes = {
|
|
notification: ImmutablePropTypes.map.isRequired,
|
|
settings: ImmutablePropTypes.map.isRequired,
|
|
};
|
|
|
|
renderFollow (notification) {
|
|
return (
|
|
<NotificationFollow
|
|
id={notification.get('id')}
|
|
account={notification.get('account')}
|
|
notification={notification}
|
|
/>
|
|
);
|
|
}
|
|
|
|
renderMention (notification) {
|
|
return (
|
|
<StatusContainer
|
|
id={notification.get('status')}
|
|
notification={notification}
|
|
withDismiss
|
|
/>
|
|
);
|
|
}
|
|
|
|
renderFavourite (notification) {
|
|
return (
|
|
<StatusContainer
|
|
id={notification.get('status')}
|
|
account={notification.get('account')}
|
|
prepend='favourite'
|
|
muted
|
|
notification={notification}
|
|
withDismiss
|
|
/>
|
|
);
|
|
}
|
|
|
|
renderReblog (notification) {
|
|
return (
|
|
<StatusContainer
|
|
id={notification.get('status')}
|
|
account={notification.get('account')}
|
|
prepend='reblog'
|
|
muted
|
|
notification={notification}
|
|
withDismiss
|
|
/>
|
|
);
|
|
}
|
|
|
|
render () {
|
|
const { notification } = this.props;
|
|
|
|
switch(notification.get('type')) {
|
|
case 'follow':
|
|
return this.renderFollow(notification);
|
|
case 'mention':
|
|
return this.renderMention(notification);
|
|
case 'favourite':
|
|
return this.renderFavourite(notification);
|
|
case 'reblog':
|
|
return this.renderReblog(notification);
|
|
}
|
|
|
|
return null;
|
|
}
|
|
|
|
}
|