mirror of
https://git.bsd.gay/fef/nyastodon.git
synced 2024-11-02 15:21:11 +01:00
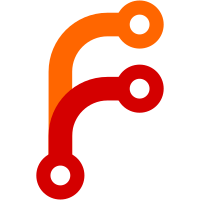
* UploadArea should only preventDefault for Escape This will make accessibility for some things less effortful, since we won't have to define a prior event handler to do whatever should be happening by default. * Remove workaround for fixed bug in SettingToggle SettingToggle was toggling itself in response to keydown of space, and then the keyup was doing it again
34 lines
1.1 KiB
JavaScript
34 lines
1.1 KiB
JavaScript
import React from 'react';
|
|
import PropTypes from 'prop-types';
|
|
import ImmutablePropTypes from 'react-immutable-proptypes';
|
|
import Toggle from 'react-toggle';
|
|
|
|
export default class SettingToggle extends React.PureComponent {
|
|
|
|
static propTypes = {
|
|
prefix: PropTypes.string,
|
|
settings: ImmutablePropTypes.map.isRequired,
|
|
settingKey: PropTypes.array.isRequired,
|
|
label: PropTypes.node.isRequired,
|
|
meta: PropTypes.node,
|
|
onChange: PropTypes.func.isRequired,
|
|
}
|
|
|
|
onChange = ({ target }) => {
|
|
this.props.onChange(this.props.settingKey, target.checked);
|
|
}
|
|
|
|
render () {
|
|
const { prefix, settings, settingKey, label, meta } = this.props;
|
|
const id = ['setting-toggle', prefix, ...settingKey].filter(Boolean).join('-');
|
|
|
|
return (
|
|
<div className='setting-toggle'>
|
|
<Toggle id={id} checked={settings.getIn(settingKey)} onChange={this.onChange} onKeyDown={this.onKeyDown} />
|
|
<label htmlFor={id} className='setting-toggle__label'>{label}</label>
|
|
{meta && <span className='setting-meta__label'>{meta}</span>}
|
|
</div>
|
|
);
|
|
}
|
|
|
|
}
|